3.4. Fourier Series#
Fourier series are a method we can use to solve inhomogeneous 2nd-order ODEs of the form
where the forcing function \(r(t)\) is periodic. This means looking like one of these examples:
# import libraries for numerical functions and plotting
import numpy as np
import matplotlib.pyplot as plt
# these lines are only for helping improve the display
import matplotlib_inline.backend_inline
matplotlib_inline.backend_inline.set_matplotlib_formats('pdf', 'png')
plt.rcParams['figure.dpi']= 300
plt.rcParams['savefig.dpi'] = 300
fig, axes = plt.subplots(3, 1)
# periodic square wave
square_wave = [1, 1, 1, 1, 0, 0, 0, 0]*10
time = np.linspace(0, 1, len(square_wave))
axes[0].plot(time, square_wave)
axes[0].grid(True)
# sin wave
time = np.linspace(0, 1, 100)
axes[1].plot(time, np.sin(time*10*np.pi))
axes[1].grid(True)
# sawtooth
y_vals = ((np.fmod(time, 2*np.pi/40)/(np.pi*2/40))*2)-1
axes[2].plot(time, y_vals)
axes[2].grid(True)
plt.tight_layout()
plt.show()
#plot(t, y); ylim([-1.5, 1.5]);
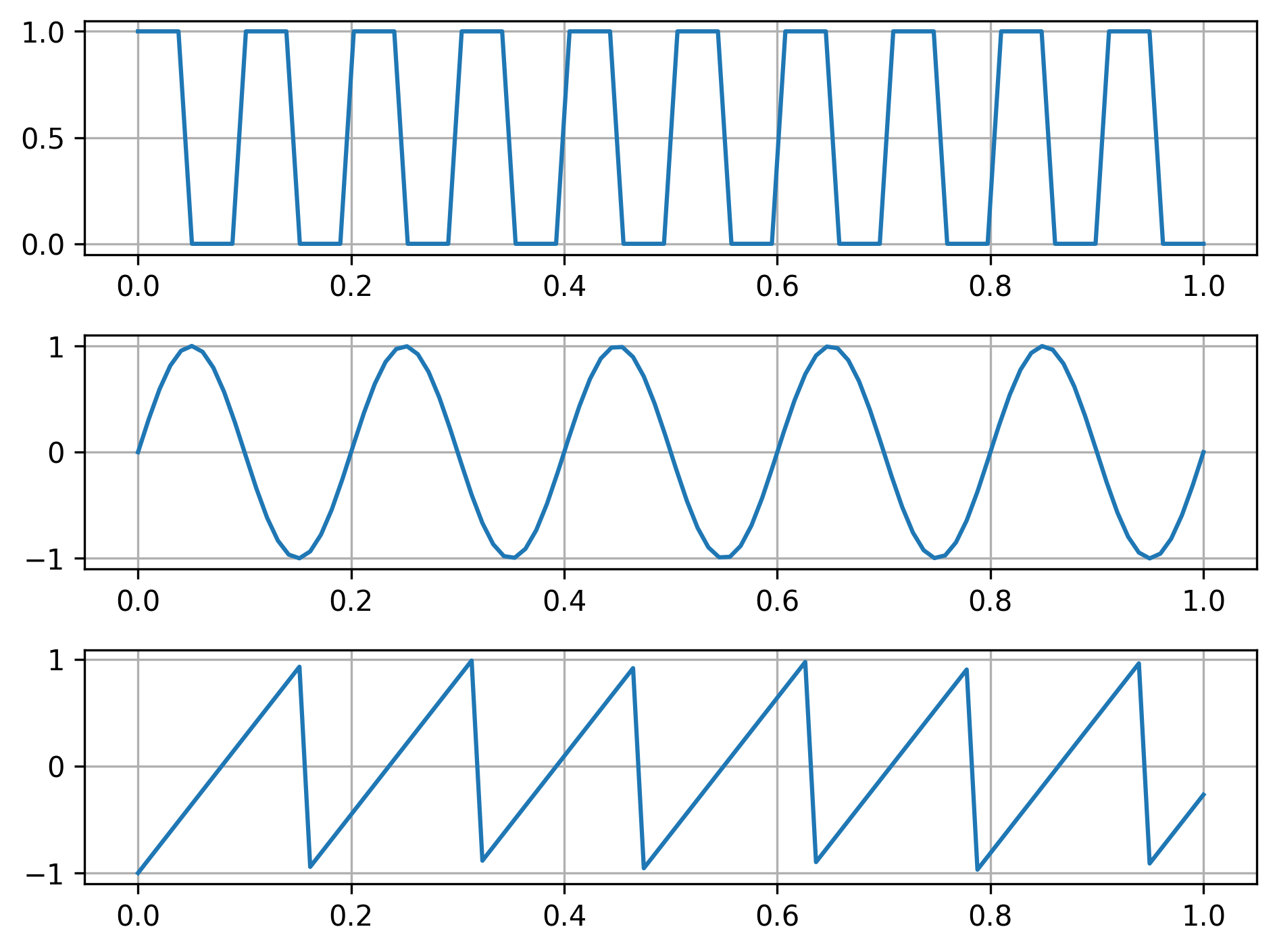
(Actually, as we’ll see later, we can use a Fourier series to represent generic forcing function!)
Fourier series have been around a while, ever since in 1790 Jean-Baptiste Joseph Fourier found that generic periodic functions could be represented by a sum of series of sin and cos functions, harmonically related by frequency.
In general, a Fourier series represents a function \(f(t)\) by
where \(a_0\), \(a_n\), and \(b_n\) are the Fourier coefficients, \(\omega = \frac{2\pi}{T}\) is the frequency of the function \(f(t)\), and \(T\) is the period. \(n\) is an integer used as an index.
3.4.1. Properties of Fourier Series#
Considering that \(n\) is an integer, and the sine and cosine components of a Fourier series share the same fundamental frequency \(\omega\), Fourier series have some useful properties:
The integral of each component trigonometric function over the period is zero:
The component trigonometric functions are orthogonal over their period:
for all values of \(n, m = 1, 2, \ldots, \infty\).
The component trigonometric functions are also orthogonal with themselves over their period:
for all values of \(n, m = 1, 2, \ldots, \infty\).
3.4.2. Fourier coefficients#
We can use the above properties to calculate the Fourier coefficients, given a periodic function \(f(t)\). First, recall
To calculate \(a_0\), integrate both sides of the equation over the period:
For the integrals of the infinite sums, recall that the integral of the sum of some functions is the same as the sum of the integrals of the functions: \(\int (a + b + c) = \int a + \int b + \int c\). That means that
since the integrals of the trigonometric functions are all zero over the period. Thus,
To calculate \(a_n\), multiply both sides of the equation by \(\cos(m \omega t)\) and integrate over the period:
Let’s take a look at each of the three integrals on the right-hand side. First,
because it just integrates cosine over the period. Skipping to the last term,
due to orthogonality. We are just left with the middle integral; let’s expand a few terms to see what that looks like:
Again, due to orthogonality, all of the terms of this infinite sum of integrals will be zero, except for the term where \(n = m\). As a result, we are left with
We can find \(b_n\) in the same way, multiplying the equation by \(\sin (m \omega t)\) and integrating over the period. The work is the same, so let’s skip that:
3.4.3. Example: periodic rectangular wave#
Let’s find the Fourier series for representing this periodic function \(f(t)\):
x = [0, 1, 1, 2, 2, 3, 3, 4]
y = [2, 2, 1, 1, 2, 2, 1, 1]
plt.plot(x, y)
plt.ylabel('f(t)')
plt.xlabel('t')
plt.ylim([0, 2.5])
plt.show()
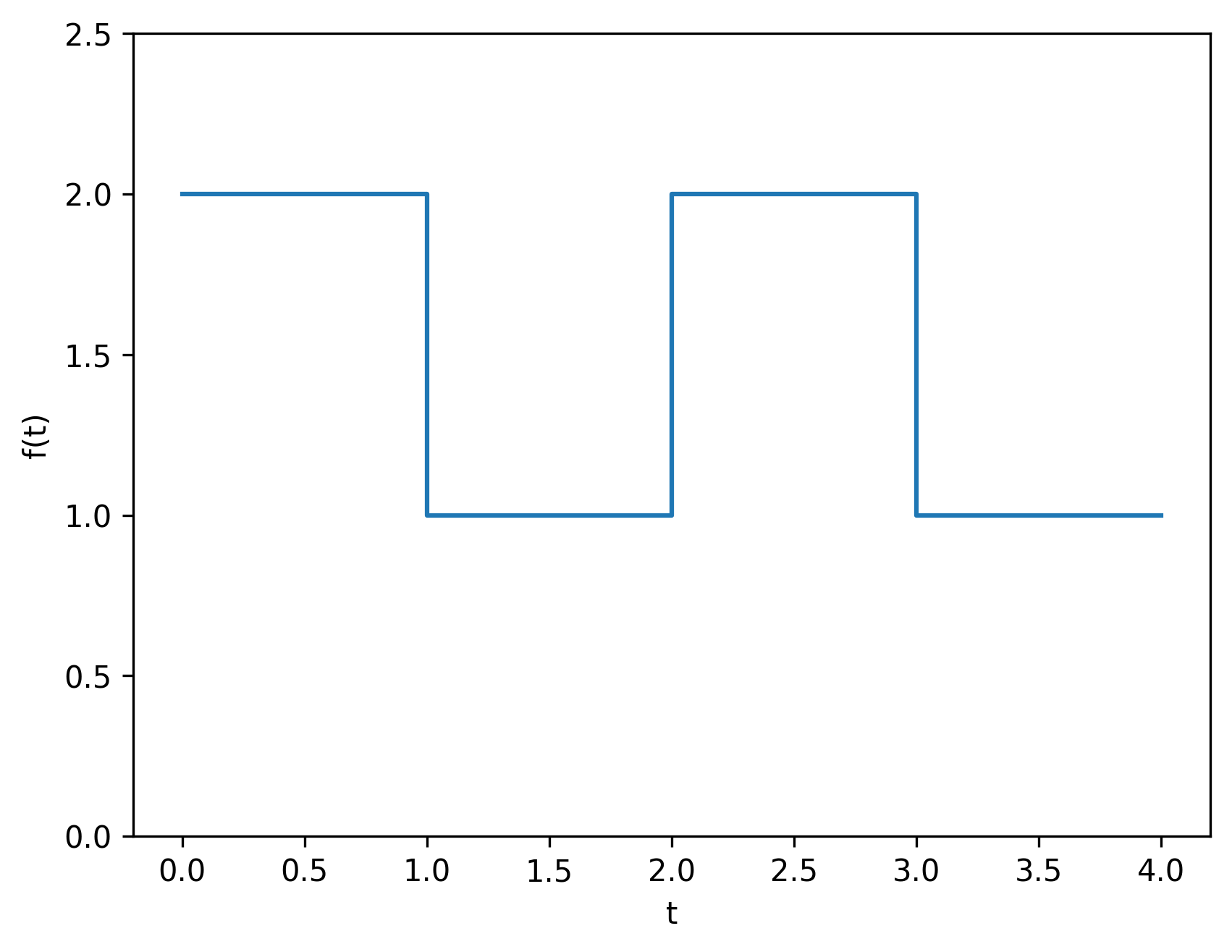
First, we need to identify the fundamental period and frequency: \(T = 2\) and then \(\omega = \frac{2\pi}{T} = \pi\). Our work is then to calculate the Fourier coefficients. Since our periodic function \(f(t)\) is not easily expressed with a function–hence the need for a Fourier series—we’ll use piecewise integration.
First, calculate \(a_0\):
Then, get \(a_n\):
Finally, we can calculate \(b_n\):
but recall that \(n = 1, 2, \ldots, \infty\). Then,
Then, our Fourier series representation for the function shown above is
Now, let’s see how whether this actually works! Let’s start with one term of the infinite sum, then gradually increase.
time = np.linspace(0, 4)
# maximum number of terms
num_max = [1, 2, 3, 5, 10, 25, 50, 250, 500]
fig, axes = plt.subplots(3, 3)
for idx, num in enumerate(num_max):
func = np.ones_like(time) * 3/2
for n in range(1, 2*num)[::2]:
func += (2 / (n*np.pi)) * np.sin(n * np.pi * time)
idy, idz = np.unravel_index(idx, (3,3))
axes[idy,idz].plot(time, func)
axes[idy,idz].set_title(f'{num} terms')
for ax in fig.get_axes():
ax.label_outer()
plt.tight_layout()
plt.show()
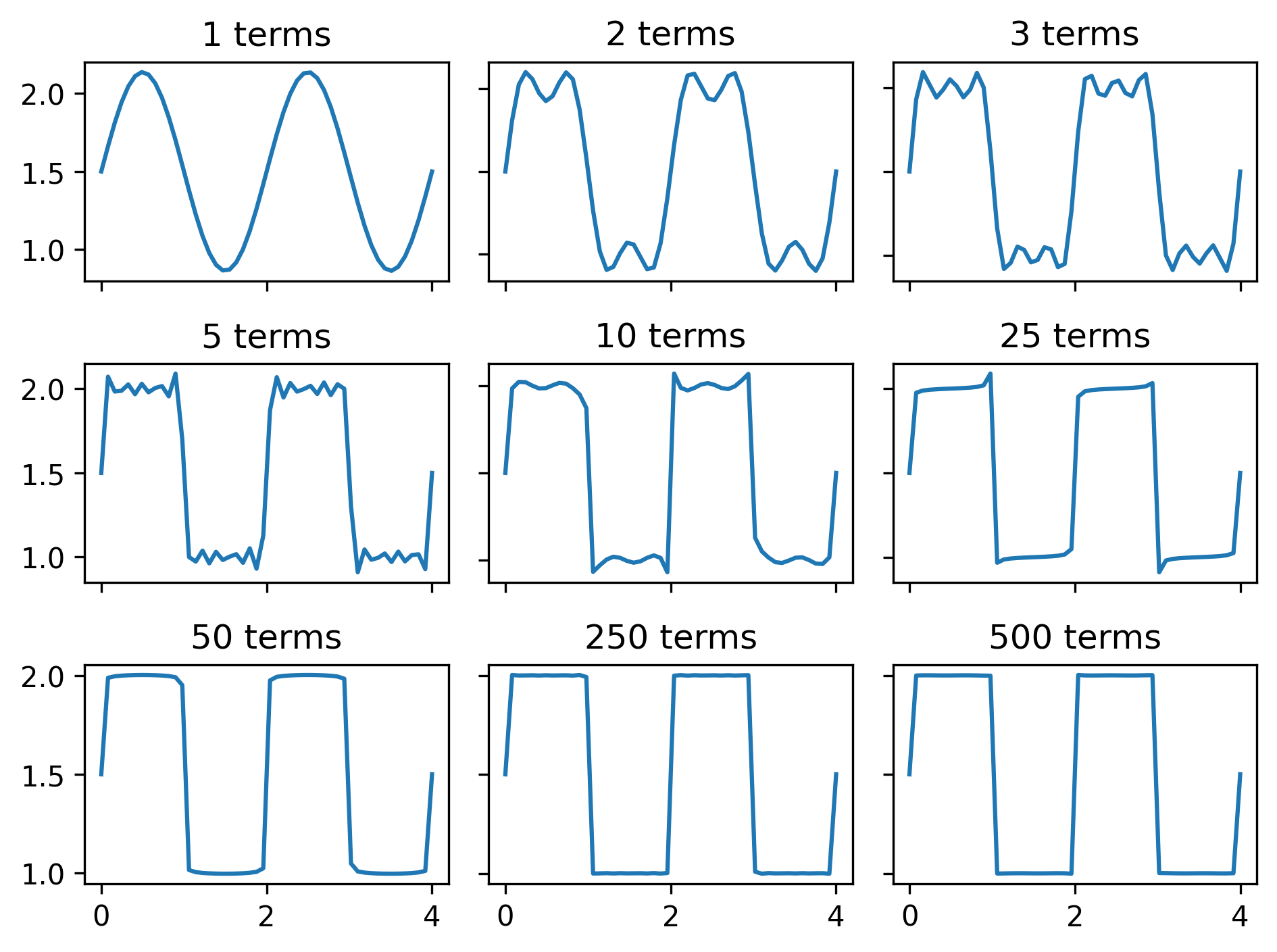
As we increase the number of terms, adding higher-frequency sine waves, we are better able to match the original rectangular wave. Notice the discrepancies that remain near the sharp corners even after the rest of the series closely resembles the function: these are known as Gibbs phenomena, caused by the Fourier series overshooting or undershooting (or “ringing”) near discontinuities.
3.4.4. Even and Odd Functions#
We can simplify our work generating a Fourier series if we can identify the given periodic function \(f(t)\) as an even function or an odd function.
Even functions are those where \(f(-x) = f(x)\).
Odd functions are those where \(f(-x) = -f(x)\).
fig, axes = plt.subplots(2, 1)
y = [-1, 0, 1, 0, -1]
x = [-2, -1, 0, 1, 2]
axes[0].plot(x, y)
axes[0].set_title('Even function')
# sets axis lines at origin
axes[0].spines['top'].set_color('none')
axes[0].spines['left'].set_position('zero')
axes[0].spines['right'].set_color('none')
axes[0].spines['bottom'].set_position('zero')
y = [0, -1, 0, 1, 0]
axes[1].plot(x,y)
axes[1].set_title('Odd function')
# sets axis lines at origin
axes[1].spines['top'].set_color('none')
axes[1].spines['left'].set_position('zero')
axes[1].spines['right'].set_color('none')
axes[1].spines['bottom'].set_position('zero')
plt.tight_layout()
plt.show()
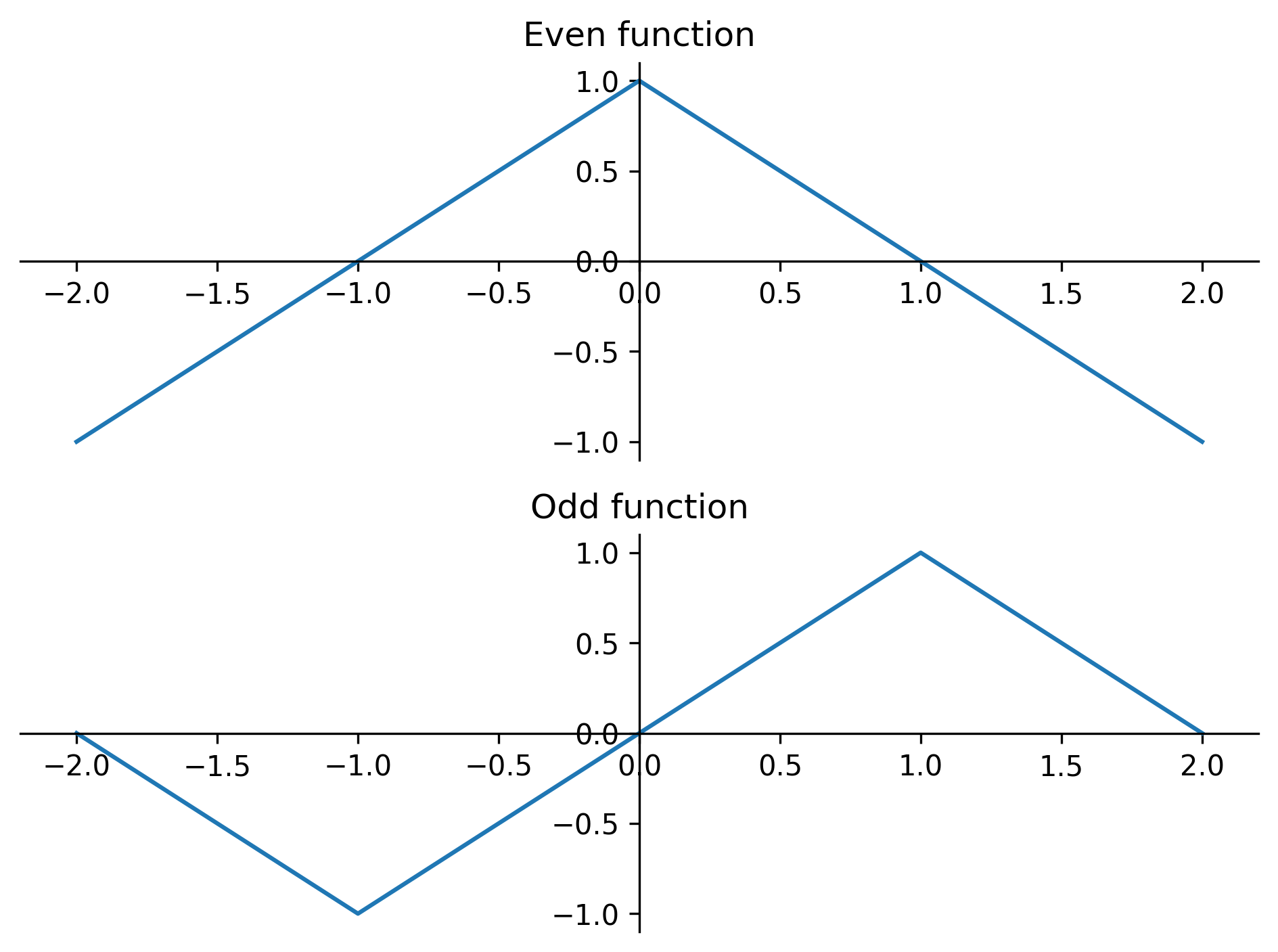
An even function’s Fourier series simplifies to a Fourier cosine series:
An odd function’s Fourier series simplifies to a Fourier sine series:
Note: not all periodic functions can be considered an even or an odd function.
3.4.5. Application: Inhomogeneous 2nd-order ODE#
One way we might use a Fourier series is to solve an inhomogeneous 2nd-order ODE, where the forcing term is given by a periodic function not easily expressed using our typical functions.
3.4.5.1. Undamped mass-spring system#
For example, let’s consider an undamped mass-spring system, where the forcing is given by a periodic rectangular wave:
where the forcing function \(f(t)\) is
time = [0, 0, 1, 1, 2, 2, 3, 3]
y = [0, 1, 1, -1, -1, 1, 1, 0]
plt.plot(time, y)
plt.title('Periodic rectangular forcing function')
ax = plt.gca()
ax.spines['top'].set_color('none')
ax.spines['bottom'].set_position('zero')
plt.show()
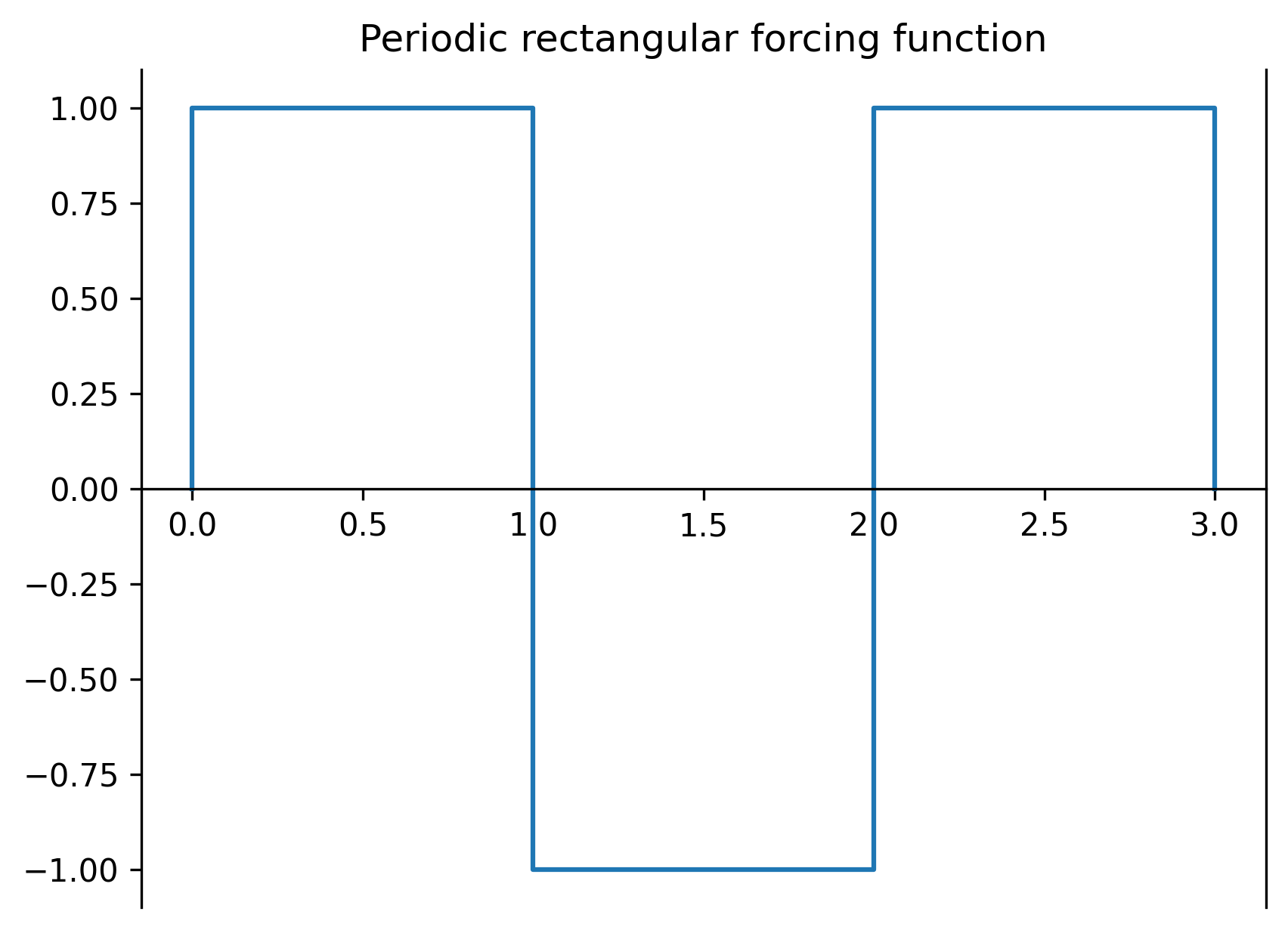
Using recognizing this as an odd function, we could construct a Fourier sine series to represent the forcing function:
Let’s confirm this works:
time = np.linspace(0, 3, 500)
func = 0
for n in range(1,1000)[::2]:
func += (4 / (n*np.pi)) * np.sin(n * np.pi * time)
plt.plot(time, func)
plt.show()
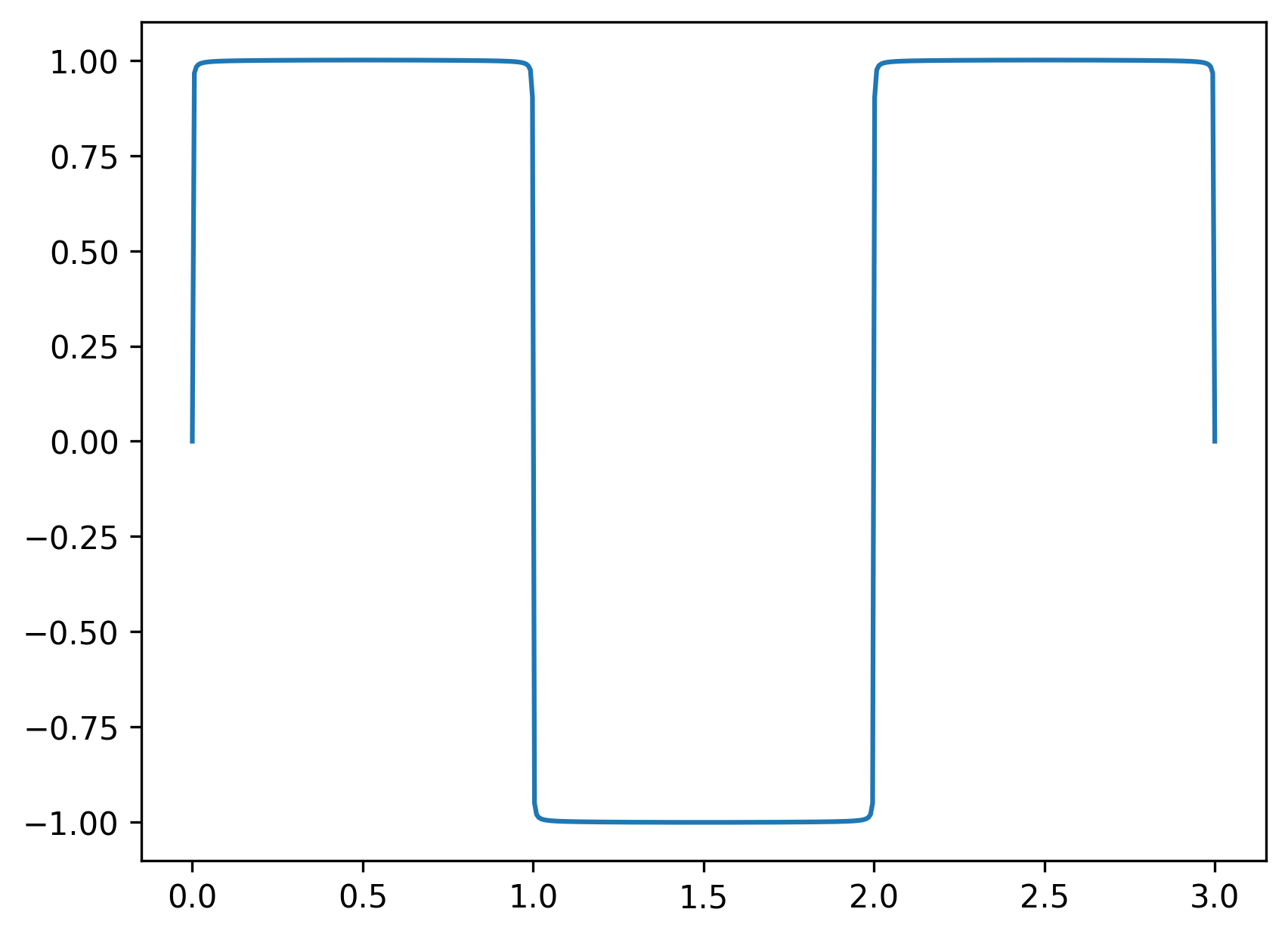
Looks good!
To find the exact solution for our displacement \(y(t)\), we can follow our usual analytical solution approach: find the homogeneous solution \(y_H\), then find the inhomogeneous solution \(y_{IH}\); the overall solution is then \(y(t) = y_H + y_{IH}\). The homogeneous solution is
We then find the inhomogeneous solution using
Solving this will give us a specific \(y_{IH, n}\); the complete inhomogeneous solution is then
Recognizing that our forcing function is sinusoidal, we should use the method of undetermined coefficients:
Inserting this into the above ODE gives
Thus, the overall solution is
3.4.5.2. Damped mass-spring system#
What about a damped mass-spring system? Recall that the homogeneous solution could take one of these three forms:
while the inhomogeneous solution, given a Fourier series forcing function, will take the form
The overall solution combines the homogenenous and inhomogeneous solutions. But, the homogeneous solution in this case is transient, because it decays to zero. On the other hand, the inhomogeneous solution remains, and is the steady-state solution.